Hello Devs,
I’m working on a project where I need to receive and answer incoming phone calls via an API and handle them with backend AI for real-time responses. Here’s a quick overview of the flow:
1. The user initiates a phone call.
2. I receive a call notification via webhook — this part is working perfectly.
3. I then need to programmatically answer the call using the /restapi/v1.0/account/~/extension/~/call-control/{session_id}/answer endpoint.
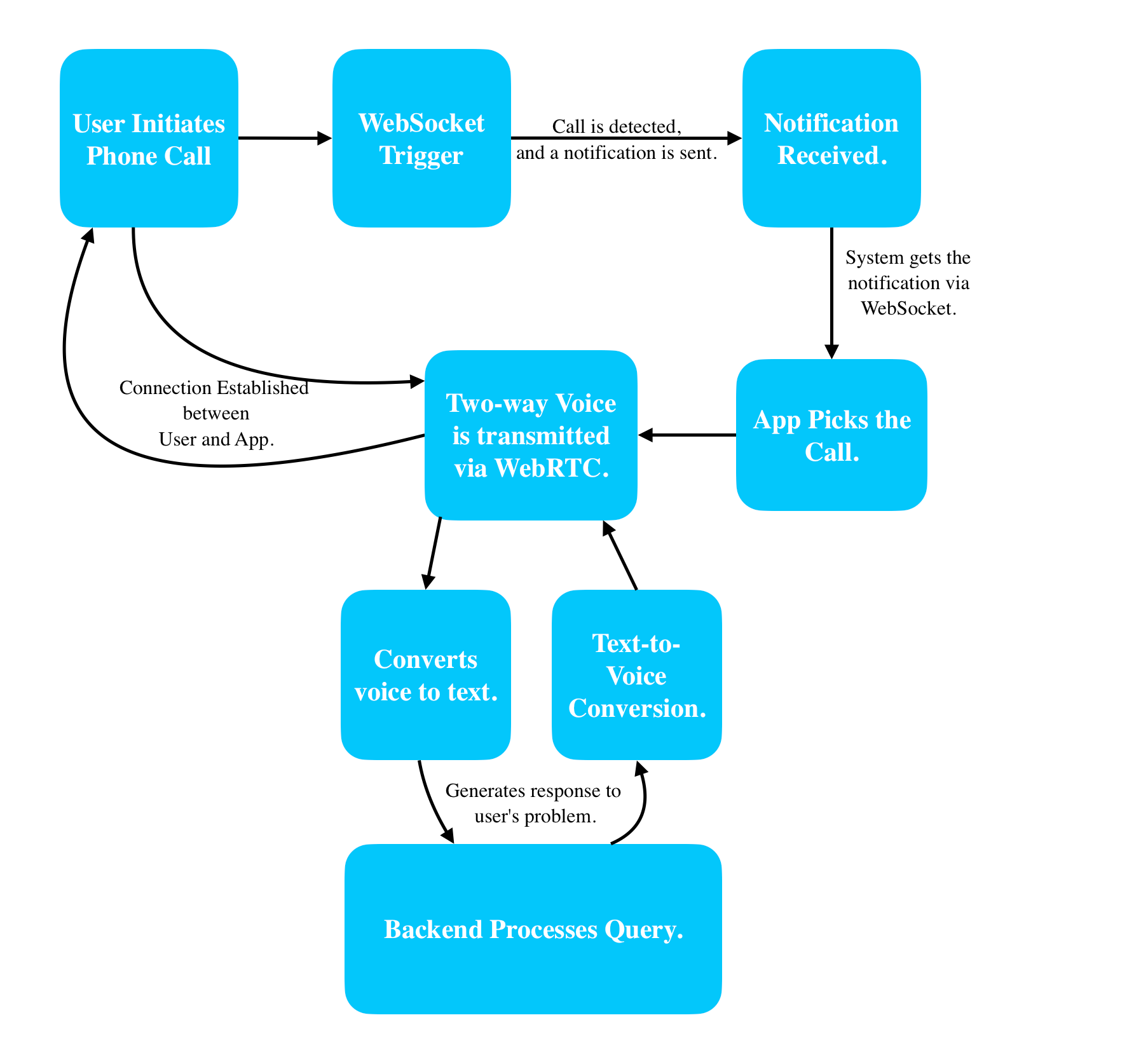
The issue is with Step 3. Despite successfully receiving the call notification, I'm unable to answer the call through this endpoint. Here’s what I’ve tried so far:
- Verified that I’m using the correct `session_id` from the webhook payload.
- Confirmed that my access token has the necessary permissions.
- Ensured that I’m using the correct API endpoint and adding necessary headers like Authorization.
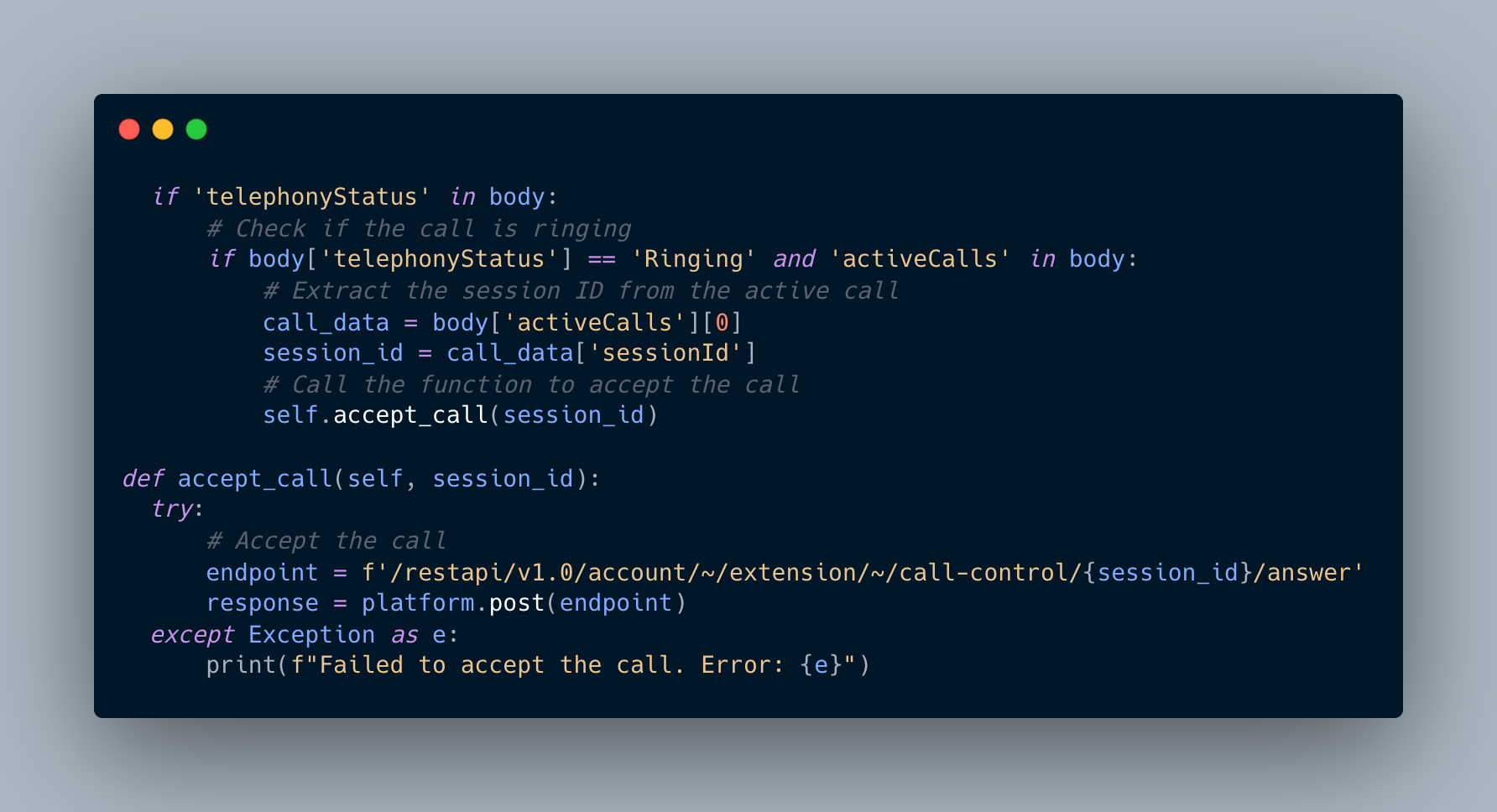
Despite these checks, the API doesn’t seem to acknowledge the answer request, and the call doesn’t get connected. My goal is to answer the call, convert the audio to text (STT), process it with AI, and respond back using text-to-speech (TTS).